mirror of
https://github.com/jech/galene.git
synced 2024-11-08 17:55:59 +01:00
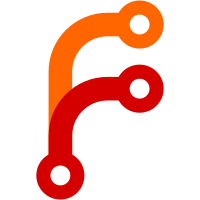
The code turned out not to port to FreeBSD. Disable the check, and assume that FreeBSD admins read the docs. Thanks to Amatis-51.
43 lines
659 B
Go
43 lines
659 B
Go
// +build linux
|
|
|
|
package limit
|
|
|
|
import (
|
|
"golang.org/x/sys/unix"
|
|
)
|
|
|
|
func Nofile(desired uint64) (uint64, error) {
|
|
var old unix.Rlimit
|
|
|
|
err := unix.Getrlimit(unix.RLIMIT_NOFILE, &old)
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
|
|
if old.Cur >= desired {
|
|
return old.Cur, nil
|
|
}
|
|
|
|
if old.Max < desired {
|
|
limit := unix.Rlimit{
|
|
Cur: desired,
|
|
Max: desired,
|
|
}
|
|
err := unix.Setrlimit(unix.RLIMIT_NOFILE, &limit)
|
|
if err == nil {
|
|
return limit.Cur, nil
|
|
}
|
|
|
|
desired = old.Max
|
|
}
|
|
|
|
limit := unix.Rlimit{
|
|
Cur: desired,
|
|
Max: old.Max,
|
|
}
|
|
err = unix.Setrlimit(unix.RLIMIT_NOFILE, &limit)
|
|
if err != nil {
|
|
return old.Cur, err
|
|
}
|
|
return limit.Cur, nil
|
|
}
|