mirror of
https://github.com/jech/galene.git
synced 2024-11-08 17:55:59 +01:00
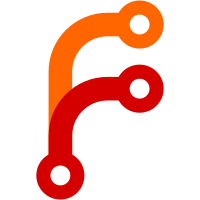
We would sometimes return nil cast to an interface with no error, which would cause the server to crash with a null dereference.
34 lines
683 B
Go
34 lines
683 B
Go
package token
|
|
|
|
import (
|
|
"errors"
|
|
"os"
|
|
)
|
|
|
|
var ErrUsernameRequired = errors.New("username required")
|
|
|
|
type Token interface {
|
|
Check(host, group string, username *string) (string, []string, error)
|
|
}
|
|
|
|
func Parse(token string, keys []map[string]interface{}) (Token, error) {
|
|
// both getStateful and parseJWT may return nil, which we
|
|
// shouldn't cast into an interface. Be very careful.
|
|
s, err1 := getStateful(token)
|
|
if err1 == nil && s != nil {
|
|
return s, nil
|
|
}
|
|
|
|
jwt, err2 := parseJWT(token, keys)
|
|
if err2 == nil && jwt != nil {
|
|
return jwt, nil
|
|
}
|
|
|
|
if err1 != nil {
|
|
return nil, err1
|
|
} else if err2 != nil {
|
|
return nil, err2
|
|
} else {
|
|
return nil, os.ErrNotExist
|
|
}
|
|
}
|